3.4.2. Beispiel zur Fourierentwicklung#
import numpy as np
from sympy import symbols, integrate, lambdify, exp, sin, cos, pi
import scipy.integrate as scint
import matplotlib.pyplot as plt
from IPython.display import Math, display
Für das Beispiel benutzen wir zwei verschiedene orthonormale Basen für den \(L_2[-1,1]\). Wir definieren das Skalarprodukt im \(L_2\) und die Norm:
t = symbols('t')
def dot(x,y):
return integrate(x*y,(t,-1,1))
def norm(x):
return dot(x,x)**(1/2)
def dotN(x,y):
xy = lambdify(t, x*y,'numpy')
return scint.quad(xy, -1, 1)[0]
def normN(x):
return dotN(x,x)**(1/2)
Legendre’sche Polynome#
Die Legendre’schen Polynome folgenden aus der Kontruktion eines ONS mit Hilfe des Verfahren nach Schmidt (vgl. vorangehendes Beispiel):
# Orthonormalbasis nach Schmidt
N = 9
yi = [t**i for i in range(N)]
xi = [yi[0]/norm(yi[0])]
for i in range(1,N):
s = 0
for j in range(i):
s += dot(yi[i],xi[j])*xi[j]
zi=yi[i]-s
xi.append(zi/norm(zi))
for xii in xi:
display(xii)
Mit Hilfe dieses ONS soll die Fourierentwicklung der Funktion
berechnet werden.
f = exp(-5*t**2)
fn = lambdify(t, f, 'numpy')
tp = np.linspace(-1,1,400)
plt.plot(tp, fn(tp))
plt.grid()
plt.show()
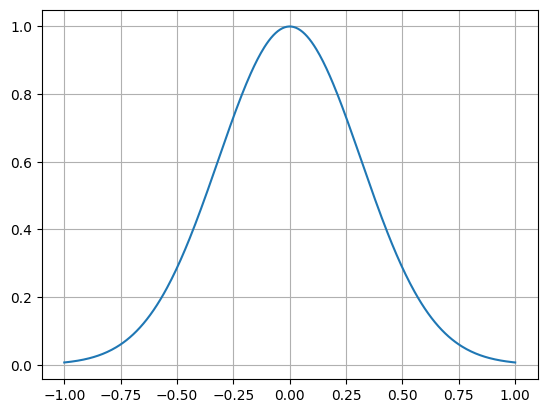
Die Fourierkoeffizienten sind gegeben durch
Die Berechnung nutzt das Skalarprodukt mit numerischer Integration.
alpha = [dotN(f, xii) for xii in xi]
alpha
[0.5596217150491691,
0.0,
-0.4411693576778274,
0.0,
0.21481237948395457,
0.0,
-0.07762200243541718,
0.0,
0.02214213441643933]
Damit erhalten wir die Fourierentwicklung von \(f(t)\)
s = 0
for alphai, xii in zip(alpha,xi):
s += alphai*xii
sn = lambdify(t, s, 'numpy')
s
tp = np.linspace(-1,1,400)
plt.plot(tp, fn(tp),label='f(t)')
plt.plot(tp, sn(tp),label='Legendre Basis')
plt.legend()
plt.grid()
plt.show()
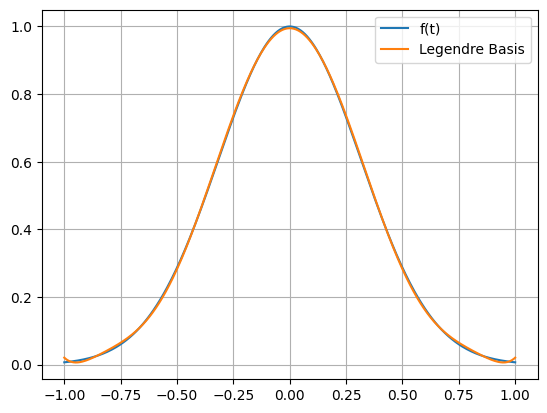
Wir überprüfen die Parsevallsche Gleichung (Vollständigkeitsrelation):
np.sum(np.array(alpha)**2)-normN(f)**2
np.float64(-2.810714668699532e-05)
Trigonometrische Funktionen#
Als zweites Set von Basisfunktionen benutzen wir \(\sin(\pi i t)\) und \(\cos(\pi i t)\).
yi = []
N = 5
yi.append(1/2)
for i in range(1,N):
yi.append(cos(pi*i*t))
yi.append(sin(pi*i*t))
print('Anzahl Basisfunktionen: '+str(len(yi)))
Anzahl Basisfunktionen: 9
Diese Funktionen sind orthogonal, entsprechend erhalten wir dieses Set unverändert aus dem Schmidtschen Orthogonalisierungsverfahren:
# Orthonormalbasis nach Schmidt
xi = [yi[0]/normN(yi[0])]
for i in range(1,2*N-1):
s = 0
for j in range(i):
s += dotN(yi[i],xi[j])*xi[j]
zi=yi[i]-s
xi.append(zi/normN(zi))
display(xi[-1])
Auf den ersten Blick sieht das Resultat nicht unverändert aus. Man beachte jedoch, dass die Koeffizienten numerisch null sind!
Für die Fourier-Koeffizienten erhalten wir:
alpha2 = [dotN(f, xii) for xii in xi]
alpha2
[0.5596217150491691,
0.48508058459128284,
0.0,
0.10914699708164805,
0.0,
0.01007774067484598,
0.0,
-0.00025517128989833646,
0.0]
und damit die Fourierreihe
s2 = 0
for alphai, xii in zip(alpha2,xi):
s2 += alphai*xii
s2n = lambdify(t, s2, 'numpy')
s2
tp = np.linspace(-1,1,400)
plt.plot(tp, fn(tp),label='f(t)')
plt.plot(tp, sn(tp),label='Legendre Basis')
plt.plot(tp, s2n(tp),label='trigonometrische Basis')
plt.legend()
plt.grid()
plt.show()
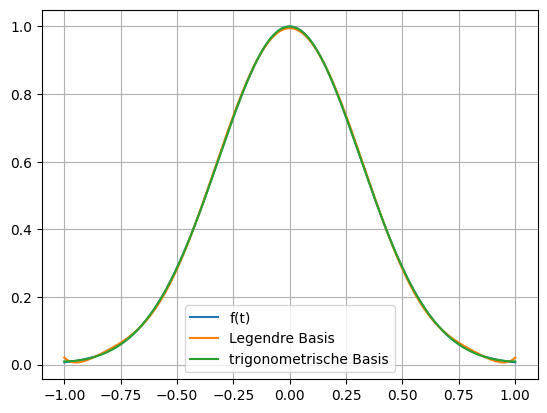
Parsevallsche Gleichung (Vollständigkeitsrelation):
np.sum(np.array(alpha2)**2)-normN(f)**2
np.float64(-4.505698534273961e-07)
Vergleich#
plt.plot(tp, sn(tp)-fn(tp),label='Legendre Basis')
plt.plot(tp, s2n(tp)-fn(tp),label='trigonometrische Basis')
plt.legend()
plt.grid()
plt.show()
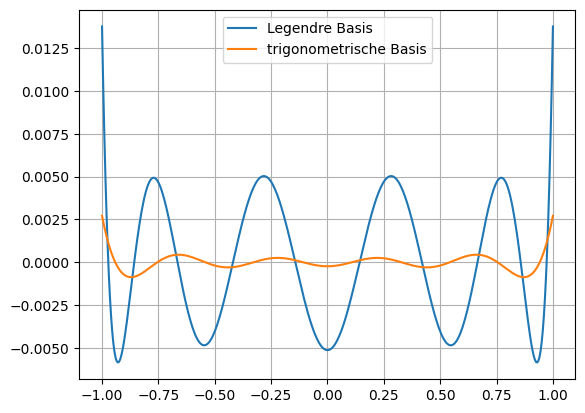