7.2.1. Beispiele zu quadratischen Formen#
import matplotlib.pyplot as plt
import numpy as np
from scipy.linalg import solve_triangular, eig, eigvals, norm, qr, svd
from sympy import symbols, lambdify
Mesh Grid für die Visualisierung
xp = np.linspace(-2,2,30)
Xp,Yp = np.meshgrid(xp,xp)
Allgemeine quadratische Form
wobei \(a\in\mathbb{R}^{n\times n}\), \(b\in\mathbb{R}^n\) und \(c\in\mathbb{R}\) sei.
def getQuadForm(a,b,c, lam = True):
x,y = symbols('x,y')
u = np.array([x,y])
expr=u@a@u+b@u+c
if lam:
return lambdify((x,y),expr)
else:
return expr
Elliptisch#
Die Matrix \(a\) muss positiv definit sein:
a = np.array([[2,1],[0,1]])
b = np.array([0,0])
c = 0
getQuadForm(a,b,c, lam = False)
plt.contour(Xp,Yp,getQuadForm(a,b,c)(Xp,Yp))
plt.gca().set_aspect(1)
plt.grid()
plt.show()
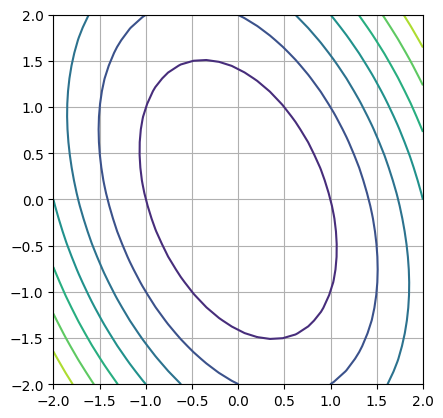
a = np.array([[-2,1],[0,-1]])
b = np.array([0,0])
c = 0
getQuadForm(a,b,c, lam = False)
eigvals(a)
array([-2.+0.j, -1.+0.j])
plt.contour(Xp,Yp,getQuadForm(a,b,c)(Xp,Yp))
plt.gca().set_aspect(1)
plt.grid()
plt.show()
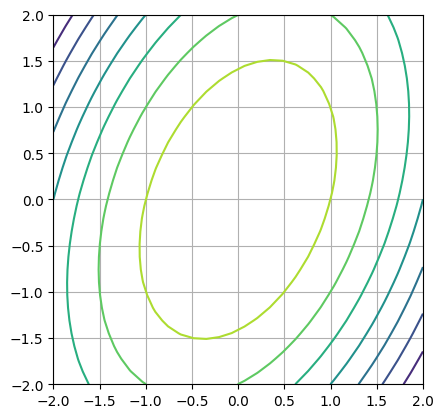
Parabolisch#
Ein kleiner Ausrutscher in die lineare Algebra: Berechnung des Kern einer Matrix#
Der Kern einer linearen Abbildung \(A: \mathbb{R}^n \to \mathbb{R}^m\) ist definiert durch die Menge der Vektoren, welche die lineare Abbildung auf den Nullvektor abbildet. Daher
Mit Hilfe der Eigenwertzerlegung#
Eine numerische Approximation des Kern einer (quadratischen) Matrix können wir mit Hilfe der zum Eigenwert \(\lambda = 0\) zugehörigen Eigenvektoren bestimmen. Das funktioniert solange die Vielfachheit eins ist. Im Fall einer Vielfachheit, ist der Eigenvektor komplexkonjugiert, womit wir mit dem Ansatz nicht alle Basisvektoren für den Nullraum erhalten. In dem Fall müsste man die Hauptvektoren bestimmen.
Als erstes erstellen wir eine 5x5 Matrix mit Rang 3:
a = np.random.randint(-9,9,size=(5,3))
# build quadratic matrix with lower rank
a = np.vstack((a.T,a[:,0]-a[:,1],a[:,2]-a[:,1])).T
a
array([[ -5, -9, 2, 4, 11],
[ 1, 6, -5, -5, -11],
[ 6, -2, -7, 8, -5],
[ -7, -8, 7, 1, 15],
[ 3, 7, 2, -4, -5]])
# Beispiel einer Matrix mit komplex konjugierten Eigenvektoren für den Eigenwert 0.
#a = np.array([[ 3, -7, 6, 10, 13],
# [ 8, 1, 8, 7, 7],
# [ 7, -8, -5, 15, 3],
# [ 2, -7, -8, 9, -1],
# [-5, -6, -8, 1, -2]])
Für die Eigenwerte bzw. Eigenvektoren zum Eigenwert 0 folgt
ew,ev = eig(a)
ns1=ev[:,(np.abs(ew)<1e-13)] # wir sind für das weitere nur am Realanteil interessiert
ns1
array([[ 0.5347377 +0.j, -0.10936594+0.j],
[-0.075137 +0.j, 0.64097877+0.j],
[-0.4596007 +0.j, -0.53161283+0.j],
[-0.5347377 +0.j, 0.10936594+0.j],
[ 0.4596007 +0.j, 0.53161283+0.j]])
a@ns1
array([[ 2.38697950e-15+0.j, 7.99360578e-15+0.j],
[ 2.77555756e-16+0.j, -4.44089210e-15+0.j],
[ 1.49880108e-15+0.j, 2.22044605e-15+0.j],
[ 8.32667268e-16+0.j, 7.10542736e-15+0.j],
[-3.33066907e-15+0.j, -6.21724894e-15+0.j]])
[norm(a@ni,np.inf) for ni in ns1.T]
[np.float64(3.1086244689504383e-15), np.float64(7.993605777301127e-15)]
Mit Hilfe der Singulärwertzerlegung#
Ein analoger Zugang, jedoch mit der Singulärwertzerlegung liefert immer eine reellwertige Lösung und den gesammten Nullraum:
atol=1e-13 # absolute Toleranz
rtol=0 # relative Toleranz
u, s, vh = svd(a) # Singulärwerte sind
tol = max(atol, rtol * s[0])
nnz = (s >= tol).sum()
ns2 = vh[nnz:].conj().T
ns2
array([[ 0.27445422, 0.54742569],
[ 0.33314841, -0.62370837],
[-0.60760263, 0.07628269],
[-0.27445422, -0.54742569],
[ 0.60760263, -0.07628269]])
a@ns2
array([[-5.55111512e-16, 3.19189120e-16],
[-1.22124533e-15, -2.31759056e-15],
[ 3.88578059e-15, 1.87350135e-15],
[-1.88737914e-15, 3.74700271e-16],
[ 4.44089210e-16, 0.00000000e+00]])
[norm(a@ni,np.inf) for ni in ns2.T]
[np.float64(5.329070518200751e-15), np.float64(2.525757381022231e-15)]
Wir zeigen, dass die Vektoren jeweilen durch Linearkombinationen der anderen darstellbar sind. Womit gezeigt ist, dass die Beschreibungen des Nullraums identisch sind.
q,r = qr(ns1,mode='economic')
r
array([[-1. +0.j, -0.32353386+0.j],
[ 0. +0.j, -0.94621659+0.j]])
sol=solve_triangular(r,q.T@ns2)
sol
array([[ 0.63476903-0.j, 0.84497342-0.j],
[ 0.5941586 -0.j, -0.8740065 -0.j]])
ns1@sol-ns2
array([[-7.21644966e-16+0.j, -2.22044605e-16+0.j],
[-4.44089210e-16+0.j, 0.00000000e+00+0.j],
[-5.55111512e-16+0.j, -1.38777878e-16+0.j],
[-2.22044605e-16+0.j, -5.55111512e-16+0.j],
[ 1.11022302e-16+0.j, -3.46944695e-16+0.j]])
Der Vorteil der Singulärwertzerlegung sieht man im Resultat der QR-Zerlegung: die beiden Vektoren sind orthogonal. Die \(R\) Matrix ist diagonal.
q,r = qr(ns2,mode='economic')
r
array([[-1.00000000e+00, 6.52929844e-18],
[ 0.00000000e+00, 1.00000000e+00]])
sol=solve_triangular(r,q.T@ns1)
sol
array([[ 0.82699945-0.j, 0.79952787-0.j],
[ 0.56220273+0.j, -0.60062899+0.j]])
ns2@sol-ns1
array([[ 6.66133815e-16+0.j, 4.30211422e-16+0.j],
[ 3.46944695e-16+0.j, 3.33066907e-16+0.j],
[ 6.66133815e-16+0.j, 4.44089210e-16+0.j],
[ 5.55111512e-16+0.j, -1.38777878e-16+0.j],
[ 0.00000000e+00+0.j, -3.33066907e-16+0.j]])
Die beiden Beschreibungen sind bis auf numerische Rundung identisch.
Zurück zur quadratischen Form#
Betrachten wir das erwähnte Beispiel: Der Nullraum ist in dem Fall offensichtlich gegeben durch \((0,1)^T\) und entsprechend kompatibel mit dem \(b\) Vektor.
a = np.array([[1,0],[0,0]])
b = np.array([0,1])
c = 0
getQuadForm(a,b,c, lam = False)
plt.contour(Xp,Yp,getQuadForm(a,b,c)(Xp,Yp))
plt.gca().set_aspect(1)
plt.grid()
plt.show()
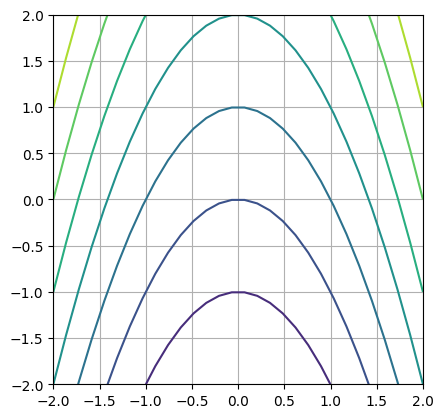
Hyperbolisch#
Wir betrachten das Beispiel:
a = np.array([[1,0],[0,-1]])
b = np.array([0,0])
c = 0
getQuadForm(a,b,c, lam = False)
plt.contour(Xp,Yp,getQuadForm(a,b,c)(Xp,Yp))
plt.gca().set_aspect(1)
plt.grid()
plt.show()
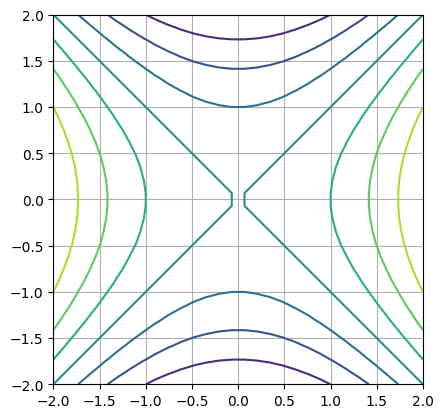